BreakDown
In this game you will use the left and right arrow keys to hit a ball and take out the bricks.
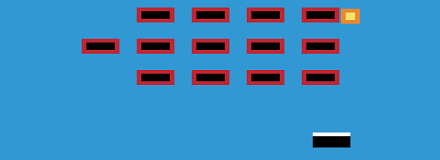
Setup our Index Page
Create a new Plunk. Edit the index.html file to look like below. The Index file is what loads our Phaser Game Engine and our BreakDown game file.
<html>
<head>
<script src="//cdn.jsdelivr.net/phaser/2.6.2/phaser.min.js"></script>
<script src="game.js"></script>
</head>
<body>
<div id="gameBoard"></div>
</body>
</html>
Create our Javascript Game file
Create a new file called game.js and copy the code below into it.
This game will have 2 states. One state is for our games Main Menu.
The other state is for our games first level.
// Game: BreakDown
// GAME STATE - MAIN MENU
// -----------------------------------------------------------------
var MainMenuState = {
preload: function() {
},
create: function() {
},
update: function() {},
// starts game at level 1
play: function() {
game.state.start('level1');
},
loadCompleted: function() {
this.create;
}
};
// GAME STATE - LEVEL 1
// -----------------------------------------------------------------
var Level1State = {
preload: function() {
// nothing here because we now load all image data in our main-menu-state6
},
create: function() {
},
update: function() {
},
gameOver: function() {
// display game over and instructions
game.add.text(50, 80, 'GAME OVER \n Restart? \n press spacebar', {
font: '50px Arial',
fill: '#FFF000'
});
// check if R key pressed
var spacebar = game.input.keyboard.addKey(Phaser.Keyboard.SPACEBAR);
spacebar.onDown.addOnce(this.restart, this);
},
// restarts the game
restart: function() {
game.state.start('level1');
}
};
// GAME - INITIALIZE
// -----------------------------------------------------------------
// Startup the Phaser Game Engine
var game = new Phaser.Game(600, 500, Phaser.AUTO, 'gameBoard');
// add game scenes ( game states ) to the phase game engine
game.state.add('mainmenu', MainMenuState);
game.state.add('level1', Level1State);
// Start Game!
game.state.start('mainmenu');
Run Your Code and check for errors.
You should get a nice black canvas
Create the Games Main Menu
Add this code to the CREATE function of the MainMenuState.
This will display our games main menu. When the user presses the spacebar,
we will load our game's Level1State into the game engine to being playing the game.
create: function() {
// display a main menu and instructions
game.add.text(50, 80, 'PONG',
{
font: '50px Arial',
fill: '#ffffff'
});
game.add.text(50, 200, 'Main Menu \n> press spacebar to start!',
{
font: '25px Arial',
fill: '#00ff2e'
});
// check if user presses spacebar
var spacebar = game.input.keyboard.addKey(Phaser.Keyboard.SPACEBAR);
// if spacebar is pressed down, call the function play
spacebar.onDown.addOnce(this.play, this);
},
Run Your Code and check for errors.
Press F12 to check console tab for any errors. You should see the games main menu. Press spacebar to start up our Level1State which will just show a black screen for now.
Load in our Games Image Data
Add this code to the PRELOAD function of the MainMenuState.
This code will load in all our image data needed for our pictures.
preload: function() {
// load in all our image data we will use during our game
// image data for our paddle
var paddleRaw = [
'22222222',
'00000000',
'00000000',
'00000000'
];
game.create.texture('paddle', paddleRaw, 6, 6, 0);
// image data for our brick
var brickRaw = [
'33333333',
'30000003',
'30000003',
'33333333'
];
game.create.texture('brick',brickRaw, 6, 6, 0);
// image data for our ball
var ballData = [
'7777',
'7887',
'7887',
'7777'
];
game.create.texture('ball', ballData, 6, 6, 0);
// the computer will take a few seconds to upload our image data into the game engine.
// when the load is complete
game.load.onLoadComplete.add(this.loadCompleted, this);
game.load.start();
},
Run Your Code and check for errors.
You should see the games main menu. Press spacebar to start up our Level1State which will just show a black screen for now. Our game image data should be ready to use now.
Level 1 Game State
Add this code to the CREATE function of the Level1State.
This code will setup our game environment, create a keyboard object we can use to capture user input,
and change the games background color.
If you want to change your games background, google 'rgb color picker'.
Use the tool to choose your color then copy and paste the RGB code (the number that looks like this "#3298d3").
// create all our game objects and setup our game environment for this scene
//---------------------------------------------------------------
// add physics to this level
game.physics.startSystem(Phaser.Physics.ARCADE);
// create a keyboard object to capture users input
this.keyboard = game.input.keyboard;
// change background color
game.stage.backgroundColor = "#F16FFF";
Run Your Code and check for errors.
Your games background should now be the color you choose.
Add our game paddle
Add this code to the CREATE function of the Level1State.
This code will create our paddle object.
// create our paddle object
//---------------------------------------------------------------
this.paddle = game.add.sprite(200, 450, 'paddle');
// add physics to our paddle object
game.physics.enable(this.paddle, Phaser.Physics.ARCADE);
// Set the pivot point to the center of the sprite object
this.paddle.anchor.setTo(0.5, 0.5);
// keep paddle from moving off the screen
this.paddle.body.collideWorldBounds=true;
// the paddle is immovable when another sprite runs into it
this.paddle.body.immovable = true;
Run Your Code and check for errors.
You should see the paddle on the screen now.
Make our Paddle move
Add this code to the UPDATE function of the Level1State.
This code will allow us to move our paddle back and forth.
// logic to move paddle back and forth
// move paddle left if arrow key down
if (this.keyboard.isDown(Phaser.Keyboard.LEFT)) {
this.paddle.body.velocity.x = -400;
}
// move paddle right if arrow key down
if (this.keyboard.isDown(Phaser.Keyboard.RIGHT)) {
this.paddle.body.velocity.x = 400;
}
Run Your Code and check for errors.
You should be able to move the paddle back and forth.
Make our Paddle move
Add this code to the UPDATE function of the Level1State.
This code will allow us to move our paddle back and forth.
// logic to move paddle back and forth
// move paddle left if arrow key down
if (this.keyboard.isDown(Phaser.Keyboard.LEFT)) {
this.paddle.body.velocity.x = -400;
}
// move paddle right if arrow key down
if (this.keyboard.isDown(Phaser.Keyboard.RIGHT)) {
this.paddle.body.velocity.x = 400;
}
Run Your Code and check for errors.
You should be able to move the paddle back and forth.
Create our Ball Object
Add this code to the CREATE function of the Level1State.
This code will create our game ball!
// create our ball object
//---------------------------------------------------------------
this.ball = game.add.sprite(10, 300, 'ball');
// add physics to our ball object
game.physics.enable(this.ball, Phaser.Physics.ARCADE);
// Set the pivot point to the center of the sprite object
this.ball.anchor.setTo(0.5, 0.5);
// keep ball from moving off the screen
this.ball.body.collideWorldBounds=true;
// add some bounce to our ball (1 means 100% of energy returned)
this.ball.body.bounce.setTo(1, 1);
// set the ball in motion
this.ball.body.velocity.x = 300;
this.ball.body.velocity.y = 150;
Run Your Code and check for errors.
You should see a ball bouncing around the screen.
Check if Ball is out of bounds
Add this code to the CREATE function of the Level1State, after our 'create ball object' code.
// check collsions on all sides except bottom
game.physics.arcade.checkCollision.down = false;
// if our ball goes out of bounds, call the gameOver function
this.ball.checkWorldBounds = true;
this.ball.events.onOutOfBounds.add(this.gameOver, this);
Run Your Code and check for errors.
You should see a ball bouncing around the screen except when it hits the bottom wall.
Check if Ball hits the paddle
Add this code to the UPDATE function of the Level1State.
This code will check if there is a collision between the ball and the paddle.
Since the paddle is immovable, the ball will just bounce off of it.
// detect collision between ball and paddle
game.physics.arcade.collide(this.ball, this.paddle);
Run Your Code and check for errors.
Use the paddle to hit the ball.
Create our Brick Wall
Add this code to the CREATE function of the Level1State.
This code will create a bunch of brick objects, lining them up on the screen using a FOR loop.
// create our brick wall
//---------------------------------------------------------------
this.NUMBER_OF_BRICKS_PER_ROW = 5;
this.NUMBER_OF_BRICKS_ROWS = 4;
// create a group of brick objects which will make up our wall
this.bricks = this.game.add.group();
// make four rows of bricks
for (var j = 0; j < this.NUMBER_OF_BRICKS_ROWS; j++) {
for (var i = 0; i < this.NUMBER_OF_BRICKS_PER_ROW; i++) {
// create a brick object and determine where to put it
var brick = this.game.add.sprite(i*70+150, j*50+100, 'brick');
// add this brick to our group of bricks so we can keep track of it
this.bricks.add(brick);
// Set bricks pivot point to its center
brick.anchor.setTo(0.5, 0.5);
// Enable physics on the object
this.game.physics.enable(brick, Phaser.Physics.ARCADE);
brick.body.immovable = true;
}
}
Run Your Code and check for errors.
You should see our brick wall now.
Detect if Ball hits Break
Add this code to the UPDATE function of the Level1State.
This code will detect when a collision between the ball and any brick in the Bricks group happens.
// detect collision between ball and any bricks in our brickS group
// if collision, call 'removeBrick' function
game.physics.arcade.collide(this.ball, this.bricks, this.removeBrick);
Add this function right after the UPDATE function of the Level1State. When a collision is detected, we will call the removeBrick function.
removeBrick: function(ball, brick) {
brick.kill();
},
Run Your Code and check for errors.
When the ball collides with a brick, the brick should disappear.
You've created the BreakDown game!
What other games can you make up using this code!?
code on plnkr